In this example we connect a HC-SR04 Ultrasonic ranging module to a Pyboard
Ultrasonic ranging module HC – SR04 provides 2cm – 400cm non-contact measurement function, the ranging accuracy can reach to 3mm. The modules includes ultrasonic transmitters, receiver and control circuit.
(1) Using IO trigger for at least 10us high level signal,
(2) The Module automatically sends eight 40 kHz and detect whether there is a pulse signal back.
(3) IF the signal back, through high level , time of high output IO duration is the time from sending ultrasonic to returning.
Test distance = (high level time×velocity of sound (340M/S) / 2
You only need to supply a short 10uS pulse to the trigger input to start the ranging, and then the module will send out an 8 cycle burst of ultrasound at 40 kHz and raise its echo. The Echo is a distance object that is pulse width and the range in proportion . You can calculate the range through the time interval between sending trigger signal and receiving echo signal.
Formula: uS / 58 = centimeters or uS / 148 =inch; or: the range = high level time * velocity (340M/S) / 2; we suggest to use over 60ms measurement cycle, in order to prevent trigger signal to the echo signal.
Parts List
Connection
Wire connecting direct as following:
3v3 Supply
Trigger Pulse Input
Echo Pulse Output
0V Ground
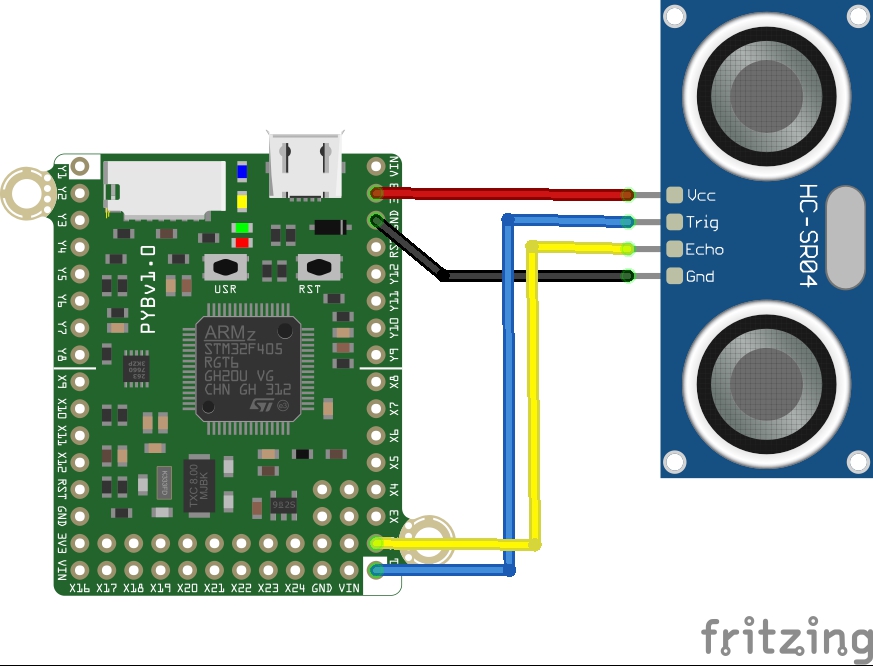
pyboard and hc-sr04
Code
2 parts to this an ultrasonic library
[codesyntax lang=”python”]
## # Ultrasonic library for MicroPython's pyboard. # Compatible with HC-SR04 and SRF04. # # Copyright 2014 - Sergio Conde Gómez <skgsergio@gmail.com> # # This program is free software: you can redistribute it and/or modify # it under the terms of the GNU General Public License as published by # the Free Software Foundation, either version 3 of the License, or # (at your option) any later version. # # This program is distributed in the hope that it will be useful, # but WITHOUT ANY WARRANTY; without even the implied warranty of # MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the # GNU General Public License for more details. # # You should have received a copy of the GNU General Public License # along with this program. If not, see <http://www.gnu.org/licenses/>. ## import pyb # Pin configuration. # WARNING: Do not use PA4-X5 or PA5-X6 as the echo pin without a 1k resistor. class Ultrasonic: def __init__(self, tPin, ePin): self.triggerPin = tPin self.echoPin = ePin # Init trigger pin (out) self.trigger = pyb.Pin(self.triggerPin) self.trigger.init(pyb.Pin.OUT_PP, pyb.Pin.PULL_NONE) self.trigger.low() # Init echo pin (in) self.echo = pyb.Pin(self.echoPin) self.echo.init(pyb.Pin.IN, pyb.Pin.PULL_NONE) def distance_in_inches(self): return (self.distance_in_cm() * 0.3937) def distance_in_cm(self): start = 0 end = 0 # Create a microseconds counter. micros = pyb.Timer(2, prescaler=83, period=0x3fffffff) micros.counter(0) # Send a 10us pulse. self.trigger.high() pyb.udelay(10) self.trigger.low() # Wait 'till whe pulse starts. while self.echo.value() == 0: start = micros.counter() # Wait 'till the pulse is gone. while self.echo.value() == 1: end = micros.counter() # Deinit the microseconds counter micros.deinit() # Calc the duration of the recieved pulse, divide the result by # 2 (round-trip) and divide it by 29 (the speed of sound is # 340 m/s and that is 29 us/cm). dist_in_cm = ((end - start) / 2) / 29 return dist_in_cm
[/codesyntax]
Now you copy that file to your Pyboard and then modify main.py as follows
[codesyntax lang=”python”]
import pyb import ultrasonic # setting pins to accomodate Ultrasonic Sensor (HC-SR04) sensor1_trigPin = pyb.Pin.board.X1 sensor1_echoPin = pyb.Pin.board.X2 # sensor needs 5V and ground to be connected to pyboard's ground # creating two Ultrasonic Objects using the above pin config sensor1 = ultrasonic.Ultrasonic(sensor1_trigPin, sensor1_echoPin) # using USR switch to print the sensor's values when pressed switch = pyb.Switch() # function that prints each sensor's distance def print_sensor_values(): # get sensor1's distance in cm distance1 = sensor1.distance_in_cm() print("Sensor1 (Metric System)", distance1, "cm") # prints values every second while True: print_sensor_values() pyb.delay(1000)
[/codesyntax]
Testing
Open up the REPL window. Here is what I saw
Sensor1 (Metric System) 0.3965517 cm
Sensor1 (Metric System) 0.3793103 cm